What are Experience Fragments in AEM?
Experience Fragments are reusable groups of content elements (like images, text, and even dynamic components) that can be utilized across multiple channels, including websites, mobile apps, and even third-party platforms. They offer a consistent way to manage and distribute content from a single source.
When integrating with React, Experience Fragments serve as content templates that can be dynamically fetched and rendered, allowing for a seamless experience between AEM’s back-end and React’s dynamic front-end.
API Integration: The Bridge Between AEM and React.
To leverage AEM Experience Fragments within a ReactJS application, the integration relies heavily on API interactions. Typically, AEM's Content Services or Sling Model Exporter is used to expose the fragment content as JSON that can be consumed by React.
- Expose Experience Fragments via AEM’s Content Services
- In AEM, navigate to Experience Fragments, create or reuse an existing fragment, and configure the content as needed.
- Ensure the fragment is exposed through AEM’s RESTful APIs by configuring the Sling Model Exporter or directly exposing a page-level model.
- Use OAuth or API keys to secure API access to your fragments, ensuring data privacy and security.
- Fetching Data in ReactJS
Once your Experience Fragment is exposed as a REST endpoint, you can fetch the content in your React application by using above (dummy)code :
useEffect(() => {
const fetchFragment = async () => {
const response = await fetch('https://your-aem-instance.com/path/to/experience-fragment.model.json');
const fragmentData = await response.json();
setFragmentContent(fragmentData);
};
fetchFragment();
}, []);
This ensures that your React app dynamically pulls content from AEM, allowing for live updates without redeploying the front-end.
- Rendering Experience Fragments Dynamically
Experience Fragments are often modular, so rendering them dynamically in React requires a flexible component-based approach. A best practice is to map the JSON structure from AEM to React components :
const ExperienceFragment = ({ data }) => {
return (
<div className="experience-fragment">
<h1 style={{ color: data.textColor }}>{data.title}</h1>
<img src={data.bannerImage} alt={data.title} />
<p>{data.description}</p>
<a href={data.link.url}>Learn more</a>
</div>
);};
Experience Fragments Architecture:
Best Practices for API Integration:
- Caching & CDN Strategy: Leverage caching layers or CDNs to reduce API calls to AEM, especially for frequently used fragments. React’s state management or external libraries like Redux can store fetched data, minimizing redundant requests.
- Error Handling: While integrating Experience Fragments, it’s crucial to handle cases where the API may fail. Implement fallback UIs to ensure a smooth user experience.
- Data Transformation & Clean-Up: Often, the JSON format provided by AEM might need transformation to fit React’s components. Build utility functions to map and sanitize the data structure from AEM, keeping the front-end logic clean.
- Monitoring & Performance Tuning: Implement monitoring for your API calls to AEM. Tools like New Relic or Google Analytics can track performance, API latency, and user engagement metrics for your Experience Fragments. Optimize rendering to ensure smooth, lag-free user interactions in your React app.
- Advanced approach: GraphQL for Optimized Content Fetching While REST APIs are standard for fetching Experience Fragments, GraphQL offers a more efficient and flexible approach by allowing you to request only the exact data needed. AEM’s GraphQL API integration is particularly useful for complex, nested fragments.
Key Benefits of GraphQL:
- Instead of retrieving the entire Experience Fragment structure via REST, you can query specific properties, minimizing payload size.
- If your fragments contain nested content models (e.g., a banner with multiple CTAs), GraphQL can retrieve the nested objects efficiently.
- GraphQL allows multiple data requests in one query, reducing the number of API calls.
Example Query for Experience Fragment in GraphQL:
query GetExperienceFragment {
experienceFragmentByPath(_path: "/content/experience-fragments/my-fragment") {
title
text
image {
src
alt
}
cta {
label
link
}
}
}
React Integration:
Using Apollo Client or Relay in your React app can facilitate seamless GraphQL integration:
import { useQuery, gql } from '@apollo/client';
const GET_FRAGMENT = gql`
query GetExperienceFragment {
experienceFragmentByPath(_path: "/content/experience-fragments/my-fragment") {
title
text
image {
src
alt
}
cta {
label
link
}
}
}
`;
const ExperienceFragment = () => {
const { loading, error, data } = useQuery(GET_FRAGMENT);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
const { title, text, image, cta } = data.experienceFragmentByPath;
return (
<div>
<h1>{title}</h1>
<p>{text}</p>
<img src={image.src} alt={image.alt} />
<a href={cta.link}>{cta.label}</a>
</div>
);
};
Schema Generation:
The GraphQL specification provides a series of guidelines on how to create a robust API for interrogating data on a certain instance. To do this, a client must fetch the Schema, which contains all the types necessary for a query.
For Content Fragments, the GraphQL schemas (structure and types) are based on Enabled Content Fragment Models and their data types.
- A Content Fragment Model:
- The corresponding GraphQL schema:
There are multiple advanced approaches for integrating the APIs with experience fragment like already explained GraphQL approach. Another one is SSR (Server-Side Rendering)/SSG (Static Site Generation), state management, lazy loading, and CDNs.
You can create a highly scalable and performant AEM-React integration. These advanced techniques optimize content delivery, minimize API calls, improve UX, and allow for efficient handling of complex content and media.
Integrating AEM Experience Fragments with ReactJS opens a world of possibilities for developers and marketers alike. It allows content to be managed efficiently on AEM, while React provides the flexibility to deliver modern, engaging, and dynamic interfaces.
By adopting best practices in API management, security, and front-end optimization, you can create highly scalable applications that offer consistent and personalized user experiences across various digital channels. This collaboration between AEM and React empowers teams to leverage the best of both worlds—content agility with AEM and front-end performance with React.
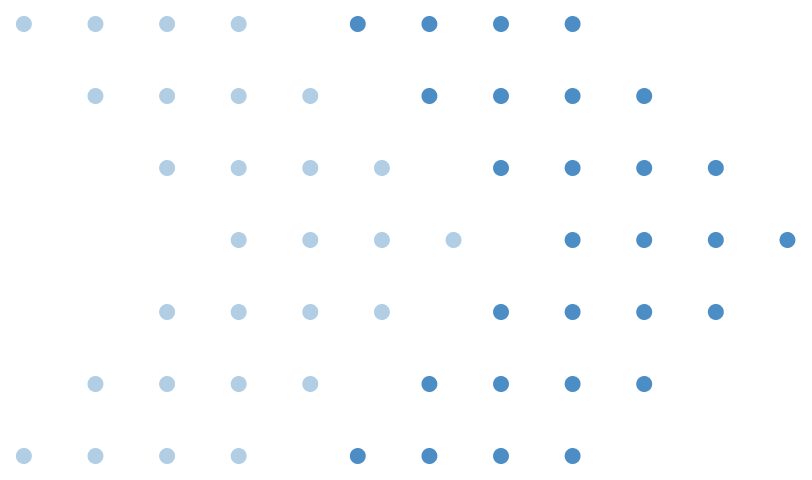